Microservices
- Service discovery in Spring Boot (Spring Cloud + Netflix Eureka)
- Circuit Breaker Pattern in Microservices (Spring BOOT + Resilience4j)
- Docker commands every developer should know
- Edge Server Pattern in Microservices (Spring Cloud Gateway)
- Monitoring Microservices (Spring Boot + Micrometer + Prometheus + Grafana)
- Spring Cloud config server setup with Git
- Distributed Tracing in Microservices (Spring Cloud Sleuth + Zipkin)
- Deploying Spring Boot microservices on Kubernetes Cluster
Mar 31, 2024
This article guides you through the deployment of two Spring Boot microservices, namely "order-service" and "inventory-service," on Kubernetes using "MiniKube".
We will establish communication between them, with "order-service" making calls to an endpoint in "inventory-service." Additionally, we will configure "order-service" to be accessible from the local machine's browser.
1) Create Spring Boot microservices
The Spring Boot microservices, "order-service" and "inventory-service," have been developed and can be found in this GitHub repository.
If you are interested in learning more about creating Spring Boot REST microservices, please refer to this or this (Reactive) link.
2) Build Docker Images
The Docker images for both "order-service" and "inventory-service" have already been generated and deployed on DockerHub, as shown below.
These images are public and can be utilized directly. For additional information on dockerizing a Spring Boot application, please consult this link.
3) Install "minikube"
To install Minikube on a Mac, install Homebrew (if not already installed). Homebrew is a package manager for macOS. You can install it by running the following command in the Terminal:
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
Use Homebrew to install Minikube:
brew install minikube
4) Start Minikube
Once Minikube is installed, you can start it using the following command:
minikube start --driver docker
This command will download the Minikube ISO, create a virtual machine, and start the Minikube cluster.
Check if Minikube and kubectl are correctly configured:
minikube status
To access the Kubernetes dashboard provided by Minikube, run:
minikube dashboard
This will open the dashboard in your default web browser.
5) Install kubectl (Optional):
Kubectl serves as a command-line utility for managing interactions with Kubernetes clusters. If it's not already installed along with Minikube, you can add it to your system using Homebrew:
brew install kubectl
Run "kubectl version" to verify that the verison you’ve installed is sufficiently up-to-date.
kubectl version
6) Create Kubernetes Deployments
Create Kubernetes Deployment YAML files for both microservices.
7) Configure Communication Between Microservices
Ensure that the "order-service" can internally communicate with the "inventory-service" by using the Kubernetes service URL: http://inventory-service.default.svc.cluster.local:8081
Create a ConfigMap using a YAML file:
8) Apply the deployments
Use the "kubectl apply" command to apply it to your Kubernetes cluster.
kubectl apply -f inventory.yml kubectl apply -f inventory-config.yml kubectl apply -f order.yml
If everything proceeded smoothly, you should observe the deployment, pods, and services actively running within the Kubernetes (K8S) dashboard, as shown in the image below.
minikube dashboard
9) Retrieve information about deployments
9.1) To retrieve information about deployments in a Kubernetes cluster, you can use the following kubectl command:
kubectl get deployments
NAME READY UP-TO-DATE AVAILABLE AGE inventory-deployment 3/3 3 3 5m11s order-deployment 3/3 3 3 5m2s
9.2) To obtain information about pods in a Kubernetes cluster along with their labels, you can use the following kubectl command:
kubectl get pods --show-labels
NAME READY STATUS RESTARTS AGE LABELS inventory-deployment-5c5cb8bf97-dpflb 1/1 Running 0 5m39s app=inventory,pod-template-hash=5c5cb8bf97 inventory-deployment-5c5cb8bf97-jjpnw 1/1 Running 0 5m39s app=inventory,pod-template-hash=5c5cb8bf97 inventory-deployment-5c5cb8bf97-tbmkz 1/1 Running 0 5m39s app=inventory,pod-template-hash=5c5cb8bf97 order-deployment-844b5dd98d-vjbzz 1/1 Running 0 5m31s app=order,pod-template-hash=844b5dd98d order-deployment-844b5dd98d-wzz86 1/1 Running 0 5m31s app=order,pod-template-hash=844b5dd98d order-deployment-844b5dd98d-x6bg9 1/1 Running 0 5m31s app=order,pod-template-hash=844b5dd98d
9.3) The "kubectl get all" command is used to retrieve information about various Kubernetes resources within the current context and namespace.
kubectl get all
NAME READY STATUS RESTARTS AGE pod/inventory-deployment-5c5cb8bf97-dpflb 1/1 Running 0 6m47s pod/inventory-deployment-5c5cb8bf97-jjpnw 1/1 Running 0 6m47s pod/inventory-deployment-5c5cb8bf97-tbmkz 1/1 Running 0 6m47s pod/order-deployment-844b5dd98d-vjbzz 1/1 Running 0 6m39s pod/order-deployment-844b5dd98d-wzz86 1/1 Running 0 6m39s pod/order-deployment-844b5dd98d-x6bg9 1/1 Running 0 6m39s NAME TYPE CLUSTER-IP EXTERNAL-IP PORT(S) AGE service/inventory-service ClusterIP 10.111.170.1958081/TCP 6m48s service/kubernetes ClusterIP 10.96.0.1 443/TCP 6m57s service/order-service NodePort 10.109.41.137 8080:65000/TCP 6m39s NAME READY UP-TO-DATE AVAILABLE AGE deployment.apps/inventory-deployment 3/3 3 3 6m48s deployment.apps/order-deployment 3/3 3 3 6m39s NAME DESIRED CURRENT READY AGE replicaset.apps/inventory-deployment-5c5cb8bf97 3 3 3 6m47s replicaset.apps/order-deployment-844b5dd98d 3 3 3 6m39s
9.4) The "kubectl describe service" command is used to obtain detailed information about a specific Kubernetes service:
kubectl describe service order-service
Name: order-service Namespace: default Labels:Annotations: Selector: app=order Type: NodePort IP Family Policy: SingleStack IP Families: IPv4 IP: 10.109.41.137 IPs: 10.109.41.137 Port: 8080/TCP TargetPort: 8080/TCP NodePort: 65000/TCP Endpoints: 172.17.0.10:8080,172.17.0.8:8080,172.17.0.9:8080 Session Affinity: None External Traffic Policy: Cluster Events:
9.5) The "kubectl logs" command is used to retrieve the logs of a specific pod in a Kubernetes cluster.
kubectl logs -f order-deployment-844b5dd98d-wzz86
9.6) The "kubectl exec" command is used to execute a command in a specific pod within a Kubernetes cluster.
kubectl exec -n default pod/inventory-deployment-5c5cb8bf97-dpflb -it -- /bin/sh
10) Access in Browser
The command "minikube service
minikube service order-service --url
http://127.0.0.1:51374 Because you are using a Docker driver on darwin, the terminal needs to be open to run it.
11) Test
You can validate this configuration by utilizing the Swagger UI of the "order-service." Make a request with an integer ID, and you should receive a response similar to the example below.
The "order-service" internally communicates with the "inventory-service" to retrieve "inventory" details.
12) Delete deployments
Below commands are used to delete all deployments, pods, and services in the "default" namespace of a Kubernetes cluster.
12.1) Delete all Deployments
This command removes all deployments in the "default" namespace.
kubectl delete --all deployments --namespace=default
12.2) Delete all Pods
This command deletes all pods in the "default" namespace.
kubectl delete --all pods --namespace=default
12.3) Delete all Services
This command removes all services in the "default" namespace.
kubectl delete --all services --namespace=default
You can locate all the source code for the Spring Boot services and Kubernetes deployment files here: GitHub.
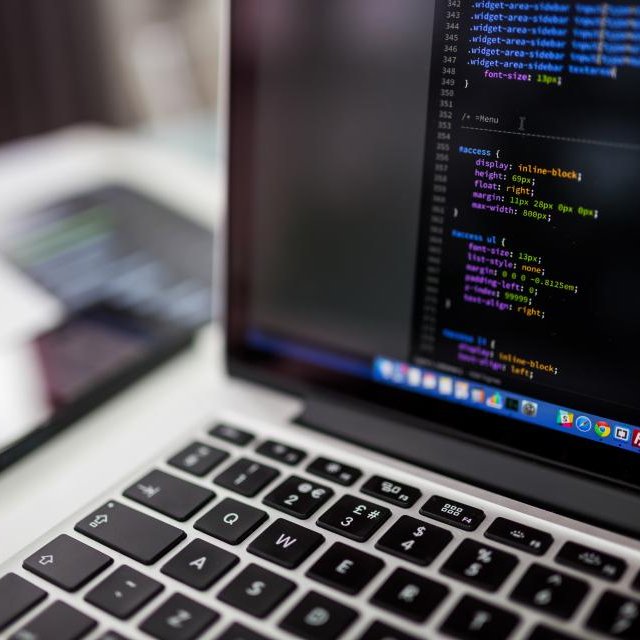
NK Chauhan
NK Chauhan is a Principal Software Engineer with one of the biggest E Commerce company in the World.
Chauhan has around 12 Yrs of experience with a focus on JVM based technologies and Big Data.
His hobbies include playing Cricket, Video Games and hanging with friends.