Spring Framework
- Profiling a Spring Boot application with Pyroscope
- Spring Boot Rest APIs with PostgreSQL (Spring Boot + Rest APIs)
- Spring's transaction management with the @Transactional annotation
- Dockerize Spring Boot app and Push image to DockerHub (Spring Boot + DockerHub)
- Spring Reactive with PostgreSQL (Spring Boot WebFlux + PostgreSQL)
- Spring Data Redis with RedisTemplate and CrudRepository
- Sending Emails in Spring Boot via SMTP
- Securing Server-to-Server Communication with "Spring Boot" & "OAuth 2"
- Spring Boot, Thymeleaf Hello World (Spring Boot + Thymeleaf + JS/CSS)
- Caching in Spring Boot (@Cacheable, @CacheEvict & @CachePut)
- Spring Boot Login/Logout (Spring Security + MySql + Thymeleaf)
Mar 31, 2024
In this article, we will see how to perform CRUD operations in a Spring Boot WebFlux application with a PostgreSQL database.
Why non blocking?
When a traditional (non-reactive) Spring Boot application starts, it maintains a preset thread pool to handle incoming requests.
One thread picks up a request and serves it to the end.
In this approach, if the request involves a blocking IO (heavy database call) or long processing time, then the thread remains in a waiting state until the response is sent to the client.
In the meantime, this thread cannot be used to handle other requests, resulting in a waste of resources (memory, CPU, etc.).
If the request load increases and too many threads are blocked by previous requests, new incoming requests are dropped or time out.
We can handle this issue by increasing the thread pool size, but there are limitations (each new thread consumes extra resources like memory, CPU, etc.).
What is Spring WebFlux?
Spring WebFlux was added in Spring 5 as a new reactive, fully non-blocking framework.
It internally uses the Project Reactor, thus supporting the Reactive Streams Specification, and runs on such servers as Netty.
It provides a non-blocking web stack to handle concurrency with a small number of threads and fewer hardware resources.
Spring WebFlux supports both functional endpoints (using functions to route and handle requests) and annotated controllers (like old Spring MVC).
Spring WebFlux sits parallel to the traditional web framework in Spring and doesn't necessarily replace it.
Reactor Netty is the default embedded server in the Spring Boot WebFlux starter.
Netty uses the event loop model to provide highly scalable concurrency in a reactive, asynchronous manner.
What is R2DBC?
Reactive Relational Database Connectivity (R2DBC) is a specification designed from the ground up for reactive programming with SQL databases.
Spring Data R2DBC applies familiar Spring abstractions and repository support to R2DBC.
Spring Data R2DBC is compatible with H2, MariaDB, MS SQL Server, MySQL, Postgres, Oracle, etc.
What are Mono and Flux ?
The Spring WebFlux Handler method handles the request and returns Mono or Flux as a response.
Mono and Flux are both implementations of the Publisher interface.
A Mono object can emit at most one value for the onNext() request and then terminate with the onComplete() signal. Or, in case of failure, it only emits a single onError() signal.
A Flux can emit 0—many asynchronous values, possibly infinite values for onNext() requests—and then terminate with either a completion signal or an error signal.
Implementation (Spring Boot WebFlux + PostgreSQL)
Let's create a Spring Boot WebFlux application. This application will provide REST APIs to perform non-blocking CRUD operations.
We will use the PostgreSQL database to create, retrieve, update, and delete "User" related data.
1) Database
Let's first create a database and the required tables in PostgreSQL.
CREATE DATABASE "userDb" WITH OWNER = postgres ENCODING = 'UTF8' LC_COLLATE = 'C' LC_CTYPE = 'C' TABLESPACE = pg_default CONNECTION LIMIT = -1; CREATE TABLE users ( id serial primary key, first_name varchar(50), last_name varchar(50) )
2) Dependencies
Let's create a project with spring initializr, make sure to add required dependencies as shown in the picture below:
Dependencies
The final "pom.xml" should look something like this:
3) Configuration
Let's add the required "postgresql" related properties to "application.yml".
4) Model
We are creating non-blocking "CRUD" Rest APIs for a "User." Here are the model and dto to represent it:
5) Repository
This is where Spring Data R2DBC" comes into the picture, we are creating a "UserRepository" implementing "ReactiveCrudRepository", this will save us from writing any boilerplate code for JDBC stuff.
6) Service
This is just an abstraction between the data layer and controller; note how we are implementing "update()" to avoid any unintended data loss:
7) Controller
Finally, this is the place where we are defining the rest endpoints for our service; please pay special attention to the usage of "@RequestBody" and "@PathVariable".
8) Utility
This utility class provides static methods for conversion to and from "User" and "UserDto."
9) Spring Boot
This is a standard Spring Boot driver class; all execution begins here.
10) Testing
Now that we've completed the project setup, run the application and use Swagger for testing:
Source code: GitHub
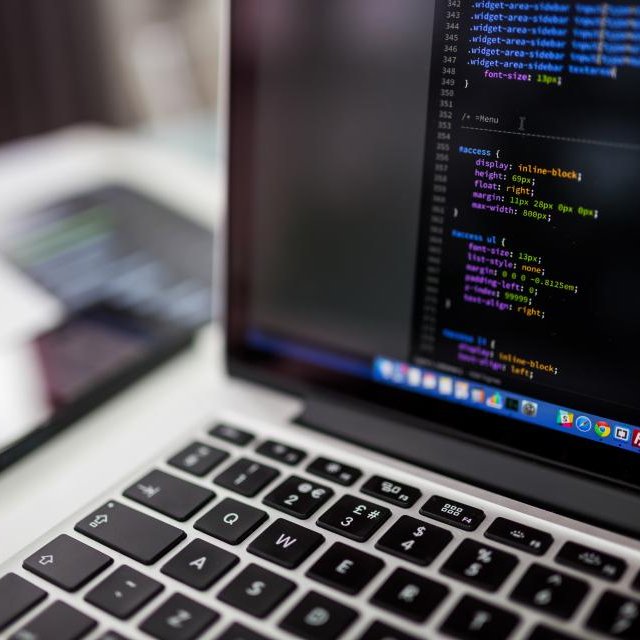
NK Chauhan
NK Chauhan is a Principal Software Engineer with one of the biggest E Commerce company in the World.
Chauhan has around 12 Yrs of experience with a focus on JVM based technologies and Big Data.
His hobbies include playing Cricket, Video Games and hanging with friends.