Spring Framework
- Profiling a Spring Boot application with Pyroscope
- Spring Boot Rest APIs with PostgreSQL (Spring Boot + Rest APIs)
- Spring's transaction management with the @Transactional annotation
- Dockerize Spring Boot app and Push image to DockerHub (Spring Boot + DockerHub)
- Spring Reactive with PostgreSQL (Spring Boot WebFlux + PostgreSQL)
- Spring Data Redis with RedisTemplate and CrudRepository
- Sending Emails in Spring Boot via SMTP
- Securing Server-to-Server Communication with "Spring Boot" & "OAuth 2"
- Spring Boot, Thymeleaf Hello World (Spring Boot + Thymeleaf + JS/CSS)
- Caching in Spring Boot (@Cacheable, @CacheEvict & @CachePut)
- Spring Boot Login/Logout (Spring Security + MySql + Thymeleaf)
Mar 31, 2024 | Updated Apr 24, 2024
Updated for: Spring Boot 3 + Java 17
What is REST ?
REST stands for representational state transfer and was created by computer scientist Roy Fielding.
REST or REpresentational State Transfer is a set of architectural constraints (not a protocol or standard) used for creating web services.
It is about creating representation (JSON, Xml, HTML etc) of object's current state and transferring that representation over a network.
A resource can be a web page, a video stream, an image, or an abstract concept, such as the list of all users in a database or the scorecard for a particular cricket match. The only constraint imposed by REST is that every resource in a system should be uniquely identifiable.
JSON is the most generally used representation format because it's language-agnostic as well as readable by both humans and machines.
The REST architectural style defines six guiding constraints.
1) Client–server architecture
In a client–server architecture, the server hosts, delivers, and manages most of the resources and services that the client requests.
It allows components to evolve independently.
2) Statelessness
The necessary state to handle the request is contained within the request itself, and no session information is retained by the server.
3) Cacheability
A response from the server must include whether it is cacheable or not and for how much time.
4) Layered system
If a proxy or load balancer is placed between the client and server, it won't affect their communications, and there won't be a need to update the client or server code.
5) Uniform interface
Individual resources should be identified in URIs, and the data should be exchanged using standard formats (JSON, XML, etc.).
A client should be able to modify or delete the resource's state with the help of the metadata attached to its representation.
Each response should include enough information to describe how to process it. For example, which parser to invoke can be specified by a media type.
Having accessed an initial URI, a client should then be able to use server-provided links to dynamically discover all the available resources it needs. Hypermedia as the engine of application state (HATEOAS)
6) Code on demand (optional)
Servers can also provide executable code to the client.
Good Practices
1) The URI of a REST API should always end with a noun. /api/users is a good example, but /api?type=users is a bad example.
A URI identifies a resource and differentiates it from others by using a name, location, or both.
2) HTTP verbs should be used to identify the action.
GET and HEAD methods should not have the significance of taking an action other than retrieval. These methods ought to be considered "safe".
On the other hand, POST, PUT, and DELETE are considered unsafe methods.
The methods GET, HEAD, PUT, DELETE, OPTIONS, and TRACE are idempotent.
Method | Details |
---|---|
GET | Request a specific resource. You can send a request body with GET, but it should be meaningless. |
HEAD | Identical to GET with the exception that the server MUST NOT return a message body in the response but still specifies the size of the response content using the Content-Length header. HTTP HEAD requests cannot have a message body. But you can still send data to the server using the URL parameters. You can send any HTTP headers with the HEAD request, just like you would for a GET request. |
POST | Add a resource to the server. It's allowed to send a POST request without a body and instead use query-string parameters. |
PUT | MUST completely replace that resource with the data enclosed in the body of the PUT. |
PATCH | Partially replace an existing resource. |
DELETE | Delete a resource from the server. DELETE can be used to send a request body, but it should be meaningless. |
CONNECT | Create an HTTP tunnel through a proxy server. The proxy server establishes a connection with the desired server on behalf of the client, and continues to proxy the TCP stream to and from the client. |
OPTIONS | Allow clients to obtain parameters and requirements for specific resources without requesting the resource. The response can include an Allow header indicating the allowed HTTP methods for this resource or various CORS (cross-origin resource sharing) headers. |
TRACE | Designed for diagnostic purposes. The server respond by echoing in its response the exact request that was received. Occasionally, this leads to the disclosure of sensitive information, such as internal authentication headers appended by reverse proxies. |
Spring Boot Rest APIs
In this section we will see how to create "Spring Boot Rest APIs" with "PostgreSQL & Spring Data JPA".
1) Dependencies
The final "pom.xml" should look something like this:
2) Properties file
Lets add required "postgresql", "connection pool" and "swagger" related properties to "application.properties".
Creating a scheme i.e. "bootpostgres" is the only thing we need to do in "PostgresDB", everything else (table creation etc.) will be automatically handled by "Spring Data JPA".
3) Model
We are creating "CRUD" Rest APIs" for an "Employee", here is the model to represent it:
4) Repository
This is where "Spring Data JPA" comes into the picture, we are creating an "EmployeeRepository" implementing "JpaRepository", this will save us from writing any boilerplate code for JDBC stuff.
Read More: Spring Data Repositories.
5) Service
This is just an abstraction between the data layer and controller; note how we are implementing "update()" to avoid any unintended data loss:
6) Controller
Finally, this is the place where we are defining the rest endpoints for our service; please take special attention to the usage of "consumes" and "produces".
7) Jpa Auditing
We are also using "Jpa Auditing" to populate "createdAt" and "updatedAt" automatically; these fields are extended to "Employee" through "AbstractEntity".
8) Spring Boot
This is plain old Spring Boot driver class; all the execution starts from here.
9) Testing
Now we are all done with our project setup, remember we have added a dependency for "springdoc-openapi-ui". This will help us in testing our end-points with an inbuilt interface.
Run the application and swagger UI for testing APIs can be accessed here: http://localhost:8080/swagger-ui.html

Profiling a Spring Boot application with Pyroscope
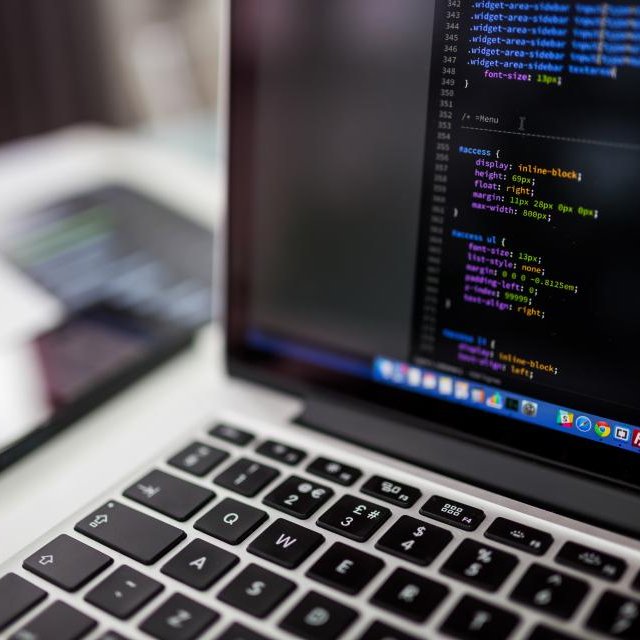
NK Chauhan
NK Chauhan is a Principal Software Engineer with one of the biggest E Commerce company in the World.
Chauhan has around 12 Yrs of experience with a focus on JVM based technologies and Big Data.
His hobbies include playing Cricket, Video Games and hanging with friends.