Spring Framework
- Profiling a Spring Boot application with Pyroscope
- Spring Boot Rest APIs with PostgreSQL (Spring Boot + Rest APIs)
- Spring's transaction management with the @Transactional annotation
- Dockerize Spring Boot app and Push image to DockerHub (Spring Boot + DockerHub)
- Spring Reactive with PostgreSQL (Spring Boot WebFlux + PostgreSQL)
- Spring Data Redis with RedisTemplate and CrudRepository
- Sending Emails in Spring Boot via SMTP
- Securing Server-to-Server Communication with "Spring Boot" & "OAuth 2"
- Spring Boot, Thymeleaf Hello World (Spring Boot + Thymeleaf + JS/CSS)
- Caching in Spring Boot (@Cacheable, @CacheEvict & @CachePut)
- Spring Boot Login/Logout (Spring Security + MySql + Thymeleaf)
Mar 31, 2024
Updated for: Spring Boot 3
This article is going to discuss how to implement "login/logout" and "authentication/authorization" in a "Spring Boot 3" application.
To demonstrate the functionality, a new "Spring Boot" application is created with the help of spring initializr.
In order to save/get user details (name, email or username, roles) the application is using the "MySql" database. The application also uses thymeleaf as a server-side template engine.
The idea is to create a custom "registration" and "login" flow based on "thymeleaf (html)" forms.
Prerequisite
This application requires an existing database in MySql; this can be achieved with the help of the below-mentioned query:
CREATE SCHEMA `spring-security-form-login` ;
Dependencies
pom.xml
The "spring-boot-starter-security" combines Spring Security dependencies, and the "spring-boot-starter-web" enables "Tomcat" as a default embedded server.
Like wise, "spring-boot-starter-data-jpa" provides the "data-jpa" dependency to efficiently connect Spring applications with relational databases.
In total, the application requires the below-mentioned dependencies:
Configuration
src/main/java/com/cb/conf/SpringSecurity.java
The "SecurityFilterChain" defines a filter chain that is capable of being matched against an HttpServletRequest.
As mentioned in the configuration below, the urls matching with "/user/**" are accessible by users having either the "USER" or "ADMIN" role, whereas the urls matching with "/admin/**" are only accessible by users having the "ADMIN" role.
On the other hand, urls beginning with "/registration/**" and "/login/**" are accessible by both authenticated and non-authenticated users, or accessible by all.
Model
src/main/java/com/cb/model/User.java
The "User" and "Role" are plain old models representing a Spring Security user and associated role in the application and database.>
src/main/java/com/cb/model/Role.java
The "Role" class has a list of users, which means a user can have multiple roles, and a role can be associated with a number of users.
DTO
src/main/java/com/cb/dto/UserDto.java
The "UserDto" class is a helper class to be used in "Html form to Controller communication" when a new user is registered in the system.
Repository
src/main/java/com/cb/repository/UserRepository.java
The "UserRepository" and "RoleRepository" are using Spring Data JPA to significantly improve the implementation of data access layers by reducing the effort to the amount that's actually needed.
src/main/java/com/cb/repository/RoleRepository.java
The "findByName" method gets the "Role" object for the associated "role" name.
Service
src/main/java/com/cb/service/UserService.java
"UserService.java" and "UserServiceImpl.java" represent an intermediate service layer between data/repository and controller.
src/main/java/com/cb/service/UserServiceImpl.java
The "saveUser()" method converts "UserDto" to "User" and saves it in the database (MySql).
src/main/java/com/cb/service/CustomUserDetailsService.java
This "CustomUserDetailsService" is a custom implementation of "UserDetailsService" and is used by Spring to get user details from the database during a login flow.
Controller
src/main/java/com/cb/controller/LoginController.java
This "LoginController" hosts "request-mappings" for "Login" and "Registration" related UI (HTML + Thymeleaf).
src/main/java/com/cb/controller/UserController.java
This "UserController" hosts a single "request-mappings" for "user-details" related UI (HTML + Thymeleaf).
Constants
src/main/java/com/cb/util/TbConstants.java
The "TbConstants.java" maintains constants used throughout the application; this helps make the application code more maintainable.
Application
src/main/java/com/cb/Application.java
This is the simple "spring boot" "main" class; all the application execution starts from here.
The only interesting thing to note is the exclusion of "SecurityAutoConfiguration.class" and "Management- WebSecurityAutoConfiguration.class". This is required to disable security auto-configuration so that we can have our own settings, like a custom login form.
HTML
src/main/resources/templates/registration.html
The "registration.html" is a simple ".html" file with "thymeleaf" template capabilities. This page contains a server-side validation-enabled "registration" form.
src/main/resources/templates/login.html
By default spring security accepts login request at "/login" and credentials in the form of "username" and "password".
src/main/resources/templates/user.html
The "thymeleaf" template engine enables us to use "sec:authentication" tags to display security-related information in HTML.
src/main/resources/application.yml
This "application.yml" file contains "db credentials," "hibernate" settings, and "thread-pool" configurations.
Testing
1) Registration
Let's run the application and start testing the functionality by registering a new user at : http://localhost:8080/registration.
2) Login
Now that a new user has been successfully registered, we can use the credentials to login at: http://localhost:8080/login.
3) Details
The user is now "logged-in" to the system with "role_user" and hence URLs accessible by "role_user" can be viewed: http://localhost:8080/user/.
3) Logout
In order to log-out from the system, we can send a get call to "http://localhost:8080/logout" i.e. click on: http://localhost:8080/logout.
Source code: GitHub
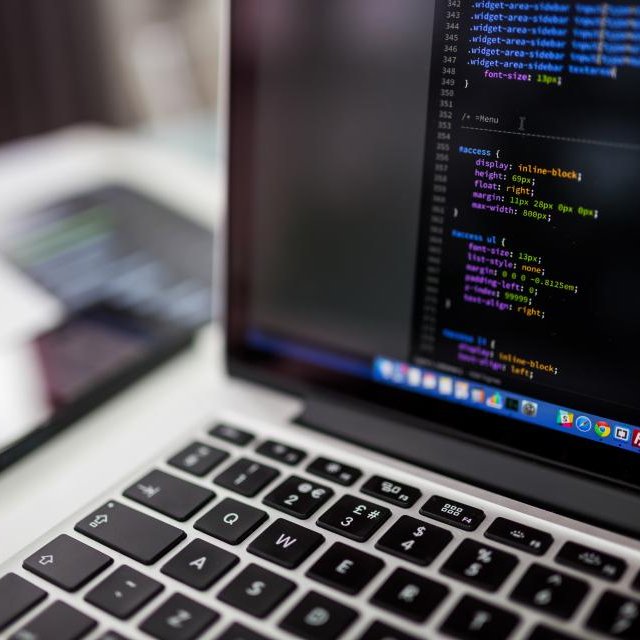
NK Chauhan
NK Chauhan is a Principal Software Engineer with one of the biggest E Commerce company in the World.
Chauhan has around 12 Yrs of experience with a focus on JVM based technologies and Big Data.
His hobbies include playing Cricket, Video Games and hanging with friends.