Front End
- Create and Run a React app and JSX Basics
Mar 31, 2024
In this article, we're going to cover, step-by-step, how to quickly and easily create and run a React app.
Prerequisite
1) You'll need to have Node >= 14.0.0 and npm >= 5.6 on your machine. More Info
2) A code editor to work with the project files: Visual Studio Code.
How to create and run a React app
1) Create a React app
"Create React App" is the best way to begin developing a new single-page React application.
npx create-react-app@5.0.1 cb-react-app cd cb-react-app npm start
The "npx" gives us the ability to use the "create-react-app" package without needing to first instal it. More Info
Open this app in "Visual Studio Code."
The "node_modules" directory contains all the React dependency packages: react, react-dom, and their transitive dependencies like webpack, babal, ESLint, etc.
The "public" folder contains static files (that don't need to be processed by webpack) such as index.html, JS files, images, etc.
The "src" folder contains actual react-related code, i.e., components, tests, CSS, etc.
The "package.json" is used to store the metadata associated with the project as well as the list of dependency packages.
To start a React project, you can simply run:
npm start
2) Add some code
Let's go ahead and delete everything in the "src" folder, so that we can add our own code to understand it better.
2.1) src/index.js
React 18 adds the new root API that comes up with the new out-of-the-box improvements.
To render a React element, first pass the DOM element to ReactDOM.createRoot(), then pass the React element to root.render().
The createRoot() method constructs a React root by passing the root element as a parameter.
Now, if you run the app with "npm start" and go to the browser at "http://localhost:3000/", you should see something like this:
2.2) Create a component
Components can be either classes or functions; let's create a function-style App component (src/App.js).
src/App.jsThe html-like syntax after return is actually JSX, and it is a syntax extension to JavaScript.
2.3) Use a component
Let's now use our newly created component "App" in "src/index.js".
After changes, our updated src/index.js will look something like this:
We have replaced plain HTML with a component. Now go to the browser at "http://localhost:3000/," and you should see something like this:
2.4) Add a CSS
Let's see how to import a CSS file (src/index.css) to style a component's elements.
Let's now use our newly created css "index.css" in src/index.js:
After changes, our updated "src/index.js" will look something like this:
Now go to the browser at "http://localhost:3000/," and you should see something like this:
3) How does this work?
The entire React app is rendered on a single HTML page, public/index.html, that too within a div with the id="root."
We don't need to change anything within the body tags of public/index.html. However, we may need to change the metadata in the head tags, i.e., title, description, favicon, etc.
4) Class Components vs. Functional Components
A functional component is just a plain JavaScript function that accepts props as an argument and returns a React element. In order to create a class component, we must extend from React Component and write a render function that returns a React element.
Functional components are stateless, as they are mainly responsible for rendering the user interface. Class components are stateful components because they implement logic and state.
React lifecycle methods like "componentDidMount" can be used inside class components but not in functional components.
Hooks can be used in functional components to make them stateful. In class components, constructors are used to store state.5) JSX Basics
5.1) Only one parent element
To render multiple elements in React, you need to wrap them within a single parent element because components can only return a single element.
Not Allowed:
Correct allowed syntax:
5.2) Use "className" instead of "class"
In JSX we use "className" instead of "class", because "class" is a JavaScript keyword.
5.3) Non JSX elements
Fundamentally, JSX element is just syntactic sugar for calling React.createElement(component, props, ...children).
With JSX
Without JSX
5.3) Dynamic JSX
Everything between {} in the JSX is just JavaScript, so you can do the following:
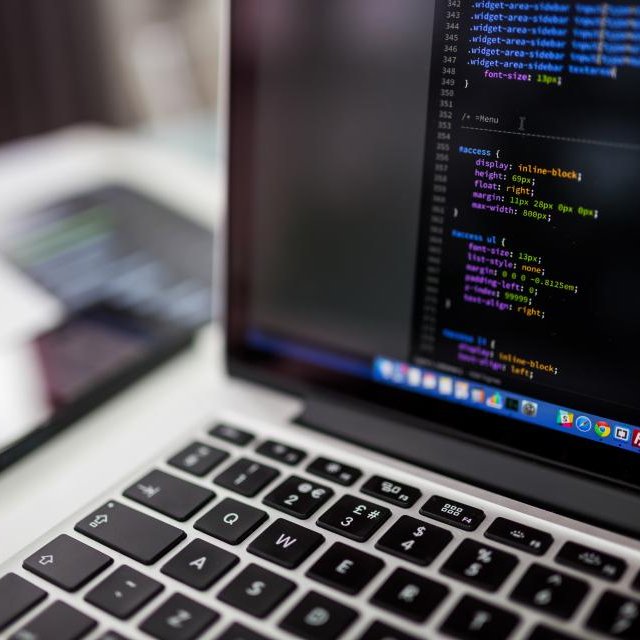
NK Chauhan
NK Chauhan is a Principal Software Engineer with one of the biggest E Commerce company in the World.
Chauhan has around 12 Yrs of experience with a focus on JVM based technologies and Big Data.
His hobbies include playing Cricket, Video Games and hanging with friends.