Microservices
- Service discovery in Spring Boot (Spring Cloud + Netflix Eureka)
- Circuit Breaker Pattern in Microservices (Spring BOOT + Resilience4j)
- Docker commands every developer should know
- Edge Server Pattern in Microservices (Spring Cloud Gateway)
- Monitoring Microservices (Spring Boot + Micrometer + Prometheus + Grafana)
- Spring Cloud config server setup with Git
- Distributed Tracing in Microservices (Spring Cloud Sleuth + Zipkin)
- Deploying Spring Boot microservices on Kubernetes Cluster
Mar 31, 2024
Here is a list of the most basic 20 Docker commands that every developer should know.
docker version
This command returns the version of both client and server ends of Docker Engine.
Version, OS, Go version, git commit and Built can be different if Docker Client and Daemon (Server) are installed separately on other machines.docker version [OPTIONS]
% docker version Client: Cloud integration: v1.0.29 Version: 20.10.20 API version: 1.41 Go version: go1.18.7 Git commit: 9fdeb9c Built: Tue Oct 18 18:20:35 2022 OS/Arch: darwin/amd64 Context: default Experimental: true Server: Docker Desktop 4.13.0 (89412) Engine: Version: 20.10.20 API version: 1.41 (minimum version 1.12) Go version: go1.18.7 Git commit: 03df974 Built: Tue Oct 18 18:18:35 2022 OS/Arch: linux/amd64 Experimental: false containerd: Version: 1.6.8 GitCommit: 9cd3357b7fd7218e4aec3eae239db1f68a5a6ec6 runc: Version: 1.1.4 GitCommit: v1.1.4-0-g5fd4c4d docker-init: Version: 0.19.0 GitCommit: de40ad0
docker info
This command provides a summarized information about the docker installation, present artifacts and kernel details of the host.
% docker info
docker build
This command is used to make Docker Images out of the written Docker files.
docker build [OPTIONS] PATH | URL
% docker build ~/code/spring-boot-docker/
This command can also be used in combination with image name and version i.e. "spring-boot-docker" and "1".
% docker build -t spring-boot-docker:1 ~/Downloads/spring-boot-docker/
docker images
This command returns all of the available images on the Docker host.
docker images [OPTIONS] [REPOSITORY[:TAG]]
% docker images REPOSITORY TAG IMAGE ID CREATED SIZE spring-boot-docker 1 da08505a6a6b 25 hours ago 489MB spring-boot-docker 1.1 da08505a6a6b 25 hours ago 489MB
The "repository-name" with and without "tag", can be used to find a particular image and see whether it exists or not.
% docker images spring-boot-docker:1.1 REPOSITORY TAG IMAGE ID CREATED SIZE spring-boot-docker 1.1 da08505a6a6b 25 hours ago 489MB
The names of the images can be identified under the REPOSITORIES column. If the same image has been built multiple times with multiple tags, it will be listed multiple times as Docker considers it different versions of the same image.
With "--no-trunc" flag, the response will not truncate the Docker Image IDs.
% docker images --no-trunc REPOSITORY TAG IMAGE ID CREATED SIZE spring-boot-docker 1 sha256:da08505a6a6bd54d61da1094a026fefb0528ece3bb3bf4f24c2b57eb74f30470 25 hours ago 489MB spring-boot-docker 1.1 sha256:da08505a6a6bd54d61da1094a026fefb0528ece3bb3bf4f24c2b57eb74f30470 25 hours ago 489MB
docker search
docker search [OPTIONS] TERM
This command offers a docker-image search on DockerHub, which works as efficiently as the GUI.
% docker search redis --limit 5 NAME DESCRIPTION STARS OFFICIAL AUTOMATED redis Redis is an open source key-value store that… 11508 [OK] bitnami/redis Bitnami Redis Docker Image 231 [OK] redis/redis-stack redis-stack installs a Redis server with add… 20 circleci/redis CircleCI images for Redis 14 [OK] redis/redis-stack-server redis-stack-server installs a Redis server w… 11
docker pull
This command pulls a target Docker Image from the desired Docker registry (public or private, by default Docker Hub).
% docker pull bitnami/redis Using default tag: latest latest: Pulling from bitnami/redis 1d8866550bdd: Pull complete 861565d18135: Pull complete Digest: sha256:719847095e818f0c1f0320f34902f0d717125458ee832743456fbd08926de657 Status: Downloaded newer image for bitnami/redis:latest docker.io/bitnami/redis:latest
docker tag
Docker Daemon uses Image IDs to primarily identify the images. Tags (a valid ASCII string) are used as a reference to point to the target image.
This command creates such tags or references that can be used by users to communicate with the daemon.
docker tag SOURCE_IMAGE[:TAG] TARGET_IMAGE[:TAG]
% docker tag da08505a6a6b spring-boot-docker:2.1
% docker tag spring-boot-docker:1 spring-boot-docker:3.1
% docker images spring-boot-docker REPOSITORY TAG IMAGE ID CREATED SIZE spring-boot-docker 1 da08505a6a6b 25 hours ago 489MB spring-boot-docker 1.1 da08505a6a6b 25 hours ago 489MB spring-boot-docker 2.1 da08505a6a6b 25 hours ago 489MB spring-boot-docker 3.1 da08505a6a6b 25 hours ago 489MB
The images are stored as repositories with tags.
If we have multiple versions of the same image, the image does not get duplicated, it just gets multiple tags (with corresponding layers if updated any) which are displayed as different images.
docker push
This command is used to push "docker images" to private or public container registries like "Docker Hub" or "jfrog".
Docker requires to follow certain image tagging rules before trying to push it.
Before pushing the image, you need to login with your repository credentials using docker login command.
% docker login -u nkchauhan003 --password-stdin < ~/docker-hub-password Login Succeeded
% docker tag spring-boot-docker:3.1 nkchauhan003/spring-boot-docker:3.1
% docker push nkchauhan003/spring-boot-docker:3.1
docker run
The docker run command first creates a writeable container layer over the specified image, and then starts it using the specified command.
A stopped container can be restarted with all its previous changes intact using docker start.
% docker run spring-boot-docker:3.1
docker stop
This docker command stop one or more running containers.
docker stop [OPTIONS] CONTAINER [CONTAINER...]
% docker stop f9f5e158ec35 f9f5e158ec35
docker ps
This command is used to view a list of all containers.
% docker ps -a CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES 8e71fb758418 spring-boot-docker:3.1 "java -jar /spring-b…" 2 minutes ago Up 2 minutes 8080/tcp vigorous_mirzakhani 53faaf02d5e3 spring-boot-docker:1 "java -jar /spring-b…" 27 hours ago Exited (130) 27 hours ago eloquent_heyrovsky 3cee1b5a878c spring-boot-docker:1 "java -jar /spring-b…" 27 hours ago Exited (130) 27 hours ago cool_haslett
docker inspect
This command invokes inspect() function of the daemon, which provides all of the data regarding the docker image in JSON array format.
% docker inspect spring-boot-docker:3.1
This command is the same for Docker Images and containers.
% docker inspect 8e71fb758418
docker rmi
This command can be used to remove one or more Docker Images addressed by their repository name, tag, or ID.
% docker rmi spring-boot-docker:1 Untagged: spring-boot-docker:1
% docker rmi spring-boot-docker:3.1 --force Untagged: spring-boot-docker:3.1
% docker rmi -f 2bf604e6f624 61f7ae0c87cd Untagged: prom/prometheus:latest
When we remove an image, it does not get deleted from the disk (otherwise, the other images would also lose the layers); just the tag gets removed from the metadata.
On the other hand, if there is only one image from a particular repository, the rmi command delete it completely.
% docker images REPOSITORY TAG IMAGE ID CREATED SIZE nkchauhan003/spring-boot-docker 3.1 da08505a6a6b 2 days ago 489MB spring-boot-docker 1.1 da08505a6a6b 2 days ago 489MB spring-boot-docker 2.1 da08505a6a6b 2 days ago 489MB spring-boot-docker 3.1 da08505a6a6b 2 days ago 489MB bitnami/redis latest 8eaa4bbee65a 3 days ago 114MB
We can remove more than one images from one or more repositories at the same time.
% docker rmi spring-boot-docker:2.1 bitnami/redis Untagged: spring-boot-docker:2.1 Untagged: bitnami/redis:latest Untagged: bitnami/redis@sha256:719847095e818f0c1f0320f34902f0d717125458ee832743456fbd08926de657 Deleted: sha256:8eaa4bbee65aa200155d30a40eb1cdb10dfb277748cd6cfba59f83fbd54aae69 Deleted: sha256:8f73e17cfed71f1e5c70f294836f17a4ced569503876f28ca543db8781d350f0 Deleted: sha256:877c59cba30815073ef56303b1b951f0e3e656a4c6170091e66bf53a1973c0c8
In the above command we removed the only bitnami/redis image.
By doing so, the layers of the image will actually be removed since there is no other bitnami/redis variant to keep these layers for.
If you do not mention the Image repository names at all, you can remove all of the images with –a flag.
docker rename
This command renames a container.
docker rename CONTAINER NEW_NAME
% docker ps -a CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES 8e71fb758418 da08505a6a6b "java -jar /spring-b…" 21 hours ago Exited (129) 19 hours ago vigorous_mirzakhani
% docker rename vigorous_mirzakhani code-burps
% docker ps -a CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES 8e71fb758418 da08505a6a6b "java -jar /spring-b…" 21 hours ago Exited (129) 19 hours ago code-burps
docker exec
This command executes the desired command on the running container.
docker exec [OPTIONS] CONTAINER NAME | ID COMMAND [ARGS]
docker run -d --name redis-cont -p 6379:6379 redis
You can only run a single command with docker exec. Piping or commands combined with && operator will not work.
% docker pull redis
% docker ps CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES 6dff4ddab591 redis "docker-entrypoint.s…" 19 seconds ago Up 19 seconds 0.0.0.0:6379->6379/tcp cb-redis
% docker exec -it cb-redis sh # redis-cli 127.0.0.1:6379> SET HELLO WORLD OK 127.0.0.1:6379> GET HELLO "WORLD" 127.0.0.1:6379>
docker stats
This command is used to get live statistics of a target container into the terminal.
% docker stats CONTAINER ID NAME CPU % MEM USAGE / LIMIT MEM % NET I/O BLOCK I/O PIDS 45c5e0e2cfd2 cb-redis-1 0.31% 2.637MiB / 3.841GiB 0.07% 726B / 0B 0B / 0B 5 2bff97798376 cb-redis 0.29% 2.504MiB / 3.841GiB 0.06% 1.16kB / 0B 0B / 0B 5
% docker stats cb-redis CONTAINER ID NAME CPU % MEM USAGE / LIMIT MEM % NET I/O BLOCK I/O PIDS 2bff97798376 cb-redis 0.30% 2.504MiB / 3.841GiB 0.06% 1.16kB / 0B 0B / 0B 5
docker cp
This command saves you from the trouble of a REST interface for sharing files from host to container and vice-versa.
docker cp /home/nkchauhan003/code/index.html cb-redis:/usr/local/code/index.html
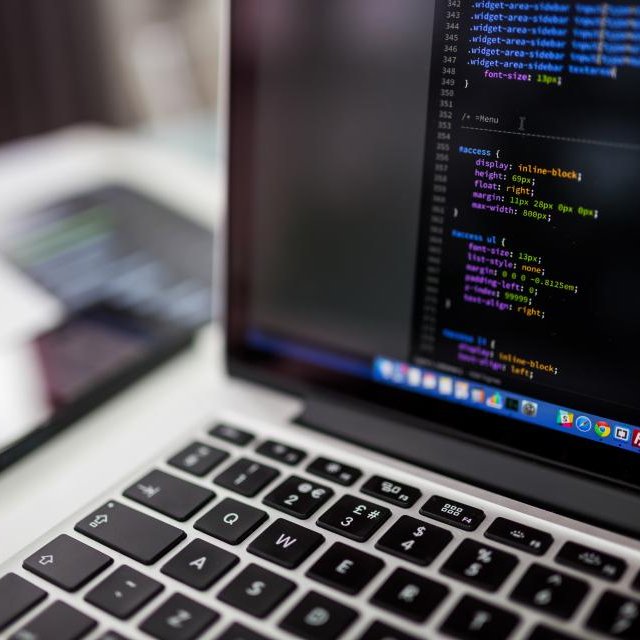
NK Chauhan
NK Chauhan is a Principal Software Engineer with one of the biggest E Commerce company in the World.
Chauhan has around 12 Yrs of experience with a focus on JVM based technologies and Big Data.
His hobbies include playing Cricket, Video Games and hanging with friends.