Microservices
- Service discovery in Spring Boot (Spring Cloud + Netflix Eureka)
- Circuit Breaker Pattern in Microservices (Spring BOOT + Resilience4j)
- Docker commands every developer should know
- Edge Server Pattern in Microservices (Spring Cloud Gateway)
- Monitoring Microservices (Spring Boot + Micrometer + Prometheus + Grafana)
- Spring Cloud config server setup with Git
- Distributed Tracing in Microservices (Spring Cloud Sleuth + Zipkin)
- Deploying Spring Boot microservices on Kubernetes Cluster
Mar 31, 2024
In this article, we will see how to use "Spring Cloud Gateway" as an edge server.
The API gateway acts as a single entry point for all clients. Some requests are simply routed to the appropriate service, while others are spread across multiple services.
All incoming requests will come to the edge server, which will route these requests based on the URL path.
Why API gateway ?
1) An API gateway helps in hiding the internal application implementation (i.e., data partitioning, service discovery, etc.) from the client.
2) The API gateway can be used to reduce the number of requests/roundtrips by enabling the clients to retrieve data from multiple services with a single round-trip.
3) API gateways can aid in the delivery of various types of data to various clients, such as HTML for HTML5/JavaScript-based UIs and REST APIs for Native Android and iPhone clients.
4) Rather than providing a one-size-fits-all API, the API gateway can provide each client with an API tailored to their specific needs.
5) The API gateway can also be used to implement security, i.e., to verify that the client is authorised to perform the request.
Implementation
We will first create two microservices, "order-service" and "product-service," where "order-service" calls a REST API exposed by "product-service."
In order to send a request to "product service," the "order-service" has a list of available instances of "product service," which is fetched periodically from the Eureka server.
We also need to set up an edge server using Spring Cloud Gateway.
A browser request to "order-service" will come through this "Edge server," and the "order-service" in turn will call the "product-service" and send back the response to the "Edge server" to send it back to the browser.
Whenever the "Edge server" routes a request to "order-service" or the "order-service" sends a request to "product-service" both of these requests are sent to instances fetched from the Eureka server.
1) Setting up "Edge server"
In order to set up a Spring Cloud Gateway as an edge server, we need to take the following steps:
1.1) Create a Spring Boot project
Let's create a project with Spring Initializr. Make sure to add the required dependencies as shown in the picture below:
The "spring-cloud-starter-netflix-eureka-client" dependency is added to enable the API gateway to locate microservice instances through Netflix Eureka.
1.2) Configuration
A route is defined by "id," "uri," and "predicate": the "id" is the name of the route, the "uri" contains the name of the service, in "Netflix Eureka" and the "predicates" is used to specify what requests this route should match.
Although not necessary, we have added routes (eureka-ui, eureka-css-js) for "eureka ui" so that we can see Netflix Eureka status through the "Edge server."
We have also exposed the "swagger UI" of "order-service" through the "Edge server" so that we can access this publicly and test the end-to-end flow of the application.
The protocol "lb://" is used to direct Spring Cloud Gateway to use the client-side load balancer to look up the destination in the discovery service.
2) Setting up "product-service"
This is a simple Spring Boot microservice with a straightforward REST API.
To know more about the interaction between "product-service", "order-service" and "Netflix Eureka," please visit: Service discovery in Spring Boot .
Complete source code for the services used in this article can be found on GitHub.
Let's start a few "product service" instances on different ports:
java -jar product-service-0.0.1-SNAPSHOT.jar --server.port=8082 & java -jar product-service-0.0.1-SNAPSHOT.jar --server.port=8083 & java -jar product-service-0.0.1-SNAPSHOT.jar --server.port=8084 &
3) Setting up "order-service"
Like "product-service," this too is a simple Spring Boot microservice with a couple of straightforward REST APIs.
To know more about the interaction between "product-service", "order-service" and "Netflix Eureka," please visit: Service discovery in Spring Boot
Complete source code for the services used in this article can be found on GitHub.
Let's start a few "order service" instances on different ports:
java -jar order-service-0.0.1-SNAPSHOT.jar --server.port=8085 & java -jar order-service-0.0.1-SNAPSHOT.jar --server.port=8086 &
4) Setting up "Netflix Eureka server"
This instance of the "Netflix Eureka server" helps in service discovery from "order-service" to "product-service" and also from the "Edge server" to "order-service". For more information, visit - How to use Netflix Eureka as a discovery service in Spring BOOT.
The "Netflix Eureka server" spring boot implementation contains a home page with a UI and we have exposed this through our "Edge server."
To view the Eureka dashboard, navigate to http://localhost:8080/eurekawebui.
Test
Now that everything is in place, we can test the overall flow with the help of the swagger UI of "order-service" exposed through the "Edge server" on: http://localhost:8080/order-service/swagger-ui.html
Source code: GitHub
Docker commands every developer should know
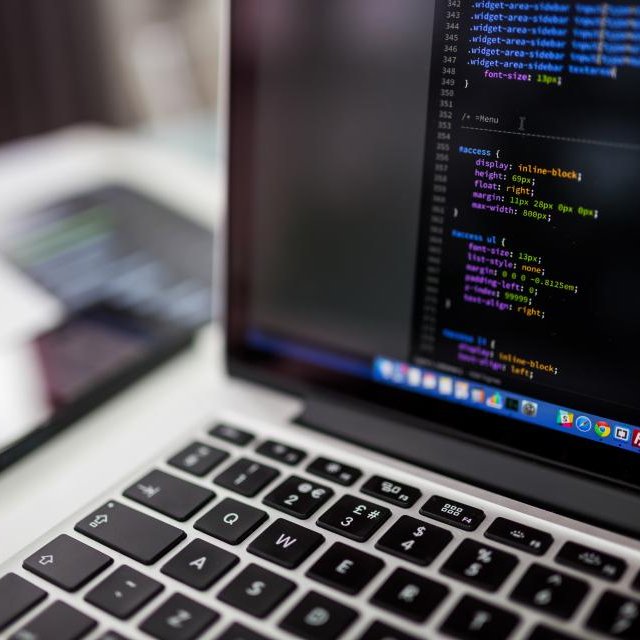
NK Chauhan
NK Chauhan is a Principal Software Engineer with one of the biggest E Commerce company in the World.
Chauhan has around 12 Yrs of experience with a focus on JVM based technologies and Big Data.
His hobbies include playing Cricket, Video Games and hanging with friends.