BigData
- Apache Kafka : Introduction and Overview
- Apache Kafka : Install Zookeeper ensemble on AWS EC2
- Apache Kafka : Developing Producer & Consumer in Java
- Elasticsearch : Introduction to Spring Data Elasticsearch
- Elasticsearch : Getting started with Elasticsearch & Kibana
- Apache Kafka : Best Practices-Topic, Partitions, Consumers and Producers
- Getting started with Spring Data MongoDB (Spring Boot + MongoDB)
- Apache Kafka : Setup Multi Broker Kafka Cluster on Amazon EC2
Mar 31, 2024
Install and start mongodb
You can install MongoDB on a Mac using Homebrew, which is a popular package manager for macOS. Here are the steps to install MongoDB on a Mac using Homebrew:
1) Open the Terminal application on your Mac.
2) Install Homebrew by running the following command in the Terminal:
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
3) Once Homebrew is installed, run the following command to update the Homebrew package database:
brew update
4) You can add the custom tap in a MacOS terminal session using:
brew tap mongodb/brew
5) Finally, run the following command to install MongoDB:
brew install mongodb-community
6) Once the installation is complete, you can start MongoDB by running the following command:
mongod --config /usr/local/etc/mongod.conf
This will start the MongoDB server. You can now connect to MongoDB using the MongoDB shell or any MongoDB client.
7) Open a new Terminal window and start mongosh by running the following command:
mongosh
Spring Data MongoDB
Spring Data MongoDB is a module of the Spring Data project that provides a set of abstractions and utility classes for working with MongoDB databases in a Spring application.
It is built on top of the MongoDB Java driver and provides a high-level, Spring-style API for interacting with MongoDB databases.
1) Connect to MongoDB
To use Spring Data MongoDB, you need to include the following dependency in your build.gradle file:
To configure a Spring Data MongoDB application using the application.yml file, you will need to define the appropriate properties for your MongoDB database connection. Here is an example configuration:
2) CRUD Operations using MongoRepository
Spring Data MongoDB provides an easy ways to perform CRUD (Create, Read, Update, Delete) operations on MongoDB databases.
2.1) Model
In Spring Data MongoDB, the @Document annotation is used to specify the name of the MongoDB collection that a POJO class maps to.
The @Id annotation is used to specify the document ID field.
When you save an instance of the Impression class to the MongoDB database, it will be stored in the "impression" collection.
If you do not specify the collection name in the @Document annotation, Spring Data MongoDB will use the name of the POJO class with the first letter in lowercase as the collection name.
In MongoDB, a collection is a grouping of MongoDB documents. It is analogous to a table in a relational database.
A collection can store any type of MongoDB document, including JSON documents. Each document in a collection can have a different structure and set of fields, which makes MongoDB a flexible and schema-less database.
Collections are created automatically when the first document is inserted into them. They can also be explicitly created using the createCollection() method. Collections can be managed using the MongoDB shell or a MongoDB client.
2.2) Repository
The MongoRepository is an interface provided by Spring Data MongoDB that defines a set of CRUD methods for interacting with a MongoDB database.
It extends the PagingAndSortingRepository and CrudRepository interfaces, which provide additional methods for pagination and sorting, and basic CRUD operations, respectively.
MongoRepository also allows you to define custom query methods by simply declaring their method signature in the repository interface.
2.3) Controller
The @RestController annotation is used to define a class as a REST controller.
The @RequestMapping annotation is used to specify the base URL path for all methods in the class.
By default, Spring Boot will automatically serialize the response object to JSON format using the Jackson JSON library, so you can return any Java object from a REST controller method and it will be automatically converted to JSON.
2.4) SpringBootApplication
@SpringBootApplication is an annotation in Spring Boot that is used to mark a Java class as the main application class for a Spring Boot application.
It is a combination of three annotations: @Configuration, @EnableAutoConfiguration, and @ComponentScan.
3) Operations using MongoTemplate
MongoTemplate is a core class in Spring Data MongoDB that provides a high-level API for interacting with MongoDB.
Testing
Swagger provides a web interface that allows you to interact with your API and test its endpoints.
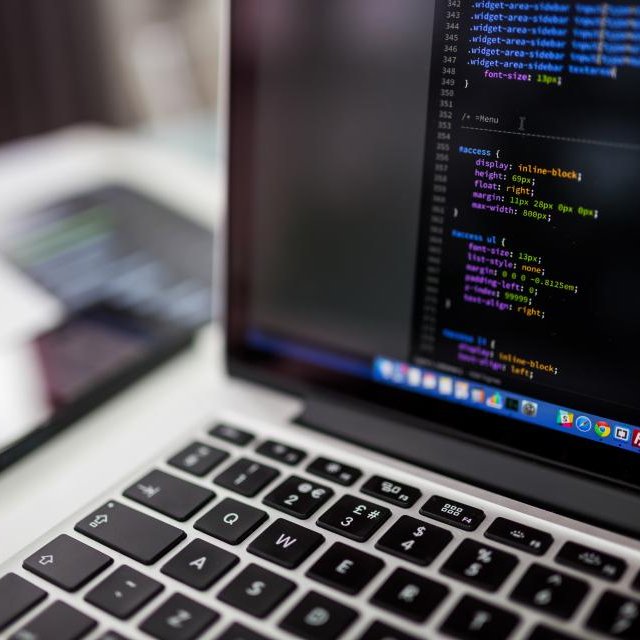
NK Chauhan
NK Chauhan is a Principal Software Engineer with one of the biggest E Commerce company in the World.
Chauhan has around 12 Yrs of experience with a focus on JVM based technologies and Big Data.
His hobbies include playing Cricket, Video Games and hanging with friends.