Core Java
- Modern Java - What’s new in Java 9 to Java 17
- Java 8 Lambdas, Functional Interface & "static" and "default" methods
- What is differences between JDK, JVM and JRE ?
- Method Reference in Java (Instance, Static, and Constructor Reference)
- What is ClassLoader in Java ?
- Object Oriented Programming (OOPs) Concept
Mar 31, 2024
Object-Oriented Programming (OOP) is a programming paradigm that revolves around the concept of "Classes and Objects."
A class is a blueprint or template that defines the structure and behavior of objects. It contains data members (fields) and methods that operate on the data.
An object is an instance of a class, representing a specific entity in the real world. Objects have state (values of their attributes) and behavior (actions they can perform).
Classes serve as a blueprint for creating objects, and objects interact with each other through method calls. Here is an example of a class and object:
In Java, OOP is a fundamental approach that allows you to model real-world entities as objects and design software with modularity, reusability, and maintainability. There are four primary OOP concepts in Java:
1) Inheritance
Inheritance allows a class (subclass/derived class) to inherit properties and behaviors from another class (superclass/base class).
It promotes code reusability and supports the "is-a" relationship between classes.
The subclass extends the superclass using the "extends" keyword, and it can override superclass methods to provide specialized behavior.
2) Polymorphism
Polymorphism allows a class to take on multiple forms, typically through method overriding and method overloading.
2.1) Method Overloading
Method overloading is the ability to define multiple methods with the same name in the same class but with different parameter lists.
The methods must have different numbers or types of parameters. The return type may be the same or different, but it alone does not determine method overloading.
The compiler determines which version of the method to call based on the number and types of arguments passed during the method invocation.
2.2) Method Overriding
Method overriding occurs when a subclass provides a specific implementation for a method that is already defined in its superclass.
The method signature (name, return type, and parameters) must be the same in both the superclass and the subclass.
The purpose of method overriding is to provide a specialized implementation of the method in the subclass, which promotes the "dynamic method dispatch" feature of polymorphism.
When an overridden method is called on an object of the subclass, the subclass's version of the method is executed, even if the reference to the object is of the superclass type.
In the example above, the Dog class overrides the makeSound() method from the Animal class, providing a specific implementation for the sound.
3) Abstraction
Abstraction allows you to hide the implementation details of an object while exposing only the essential characteristics and behaviors.
It enables you to create more manageable and understandable code by focusing on what an object does rather than how it does it.
In Java, abstraction is achieved through abstract classes and interfaces:
3.1) Abstract Classes
An abstract class is a class that cannot be instantiated directly; you cannot create objects of an abstract class.
It serves as a blueprint for other classes, allowing them to inherit its properties and methods.
Abstract classes can have both abstract (methods without a body) and concrete (methods with a body) methods.
Any class that extends an abstract class must provide implementations for all its abstract methods or be declared abstract itself.
3.2) Interfaces
An interface is a collection of abstract methods and constant variables (constants are implicitly declared as public, static, and final).
Interfaces allow classes to implement multiple behaviors without multiple inheritance conflicts.
A class implements an interface using the implements keyword and must provide concrete implementations for all the interface's methods.
4) Encapsulation
Encapsulation is the process of bundling data (attributes) and methods that operate on that data within a single unit (class).
The data members are typically declared as private to hide the internal state of an object from the outside world.
Public methods (getters and setters) are used to access and modify the object's state, enforcing controlled access to the object's data.
What is ClassLoader in Java ?
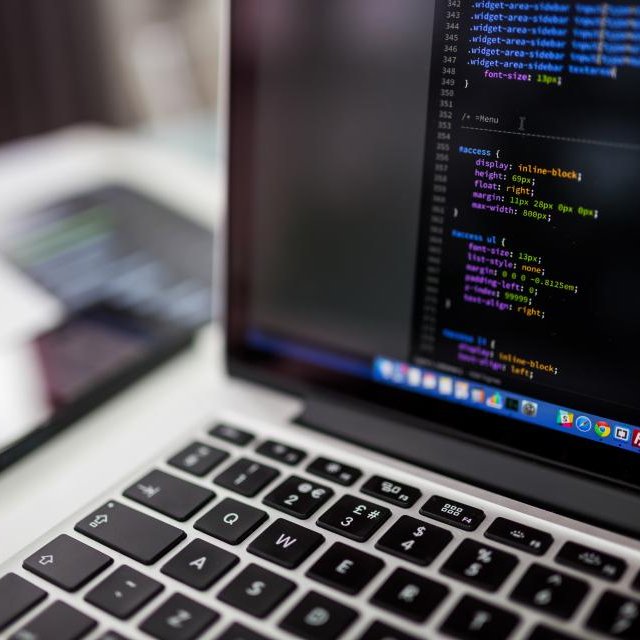
NK Chauhan
NK Chauhan is a Principal Software Engineer with one of the biggest E Commerce company in the World.
Chauhan has around 12 Yrs of experience with a focus on JVM based technologies and Big Data.
His hobbies include playing Cricket, Video Games and hanging with friends.