Core Java
- Modern Java - What’s new in Java 9 to Java 17
- Java 8 Lambdas, Functional Interface & "static" and "default" methods
- What is differences between JDK, JVM and JRE ?
- Method Reference in Java (Instance, Static, and Constructor Reference)
- What is ClassLoader in Java ?
- Object Oriented Programming (OOPs) Concept
Mar 31, 2024
Java 8: "static" and "default" methods in interface
All "interface" methods are implicitly "public" and "abstract," unless declared as "static" or "default."
As of Java 8, it is now possible to inherit concrete methods (static and default) from an interface.
The implementation class is not required to implement "static" or "default" methods.
Optionally, an implementation class can inherit "default" interface methods but not "static" interface methods.
Functional Interfaces
A functional interface is an interface that contains only one abstract method.
In this SAM (single abstract method) rule, "default," "static," and methods inherited from "Object" class do not count.
In the example above, the "int hashCode()" method is overridden from the "Object" class.
Java 8 lambdas
A "Lambda" expression is an instance of a class that implements a functional interface.
Lambdas resemble "methods," but they are instances, not "methods" or "anonymous-methods."
Using a custom Functional Interface
"Lambda" expressions only work with Functional Interfaces.
A functional interface defines the target type of a lambda expression.
The "var" keyword introduced in "Java 10" cannot be used to infer a lambda expression. ("Cannot infer type": lambda expressions require an explicit target type.)
The "java.util.function" package
The "java.util.function" package comes with a set of pre-defined functional interfaces.
1) Predicate
Predicate is a functional interface that has the functional method "boolean test(T t)". It accepts "one argument" and returns a boolean (true if the input argument matches the predicate, otherwise false).
1.1) Predicate as a method parameter
Predicates can be passed as method parameters:
1.2) Composed predicate (AND and OR)
The "default Predicate
The "default Predicate
1.3) Predicate negation
The "default Predicate
2) BiPredicate
BiPredicate is a functional interface that has the functional method "boolean test(T t, U u)". It accepts "two arguments" and returns a boolean (true if the input arguments match the predicate, otherwise false).
The "BiPredicate" interface also contains three "default" "non-abstract" methods: and(), or(), and negate().
3) Supplier
Supplier is a functional interface that has the functional method "T get()". It accepts "zero arguments" and returns a result of type T.
4) Consumer
Consumer is a functional interface that has the functional method "void accept(T t)". It accepts a single argument and returns no result.
The "default Consumer
My Name is: Leonardo DiCaprio My Name is: LEONARDO DICAPRIO
5) BiConsumer
BiConsumer is a functional interface that has the functional method "void accept(T t, U u)". It accepts two arguments and returns no result.
The "default BiConsumer
My Name is 'Leonardo DiCaprio', I am '32'. My Name is 'LEONARDO DICAPRIO', I am '32'.
6) Function
Function is a functional interface that has the functional method "R apply(T t)". It accepts one argument and returns one result.
The "default
It returns a composed function that first applies the before function to its input, and then applies this function to the result.
The "default
It returns a composed function that first applies this function to its input, and then applies the after function to the result.
7) BiFunction
BiFunction
The "default
It returns a composed function that first applies this function to its input, and then applies the after function to the result.
8) UnaryOperator
UnaryOperator
UnaryOperator represents an operation on a single operand that produces a result of the same type as its operand.
This is a specialization of Function
The "static
9) BinaryOperator
BinaryOperator
It represents an operation upon two operands of the same type, producing a result of the same type as the operands.
This is a specialization of BiFunction
The "static
The "static
Lambda Benefits
1) A lambda helps in creating a function without belonging to any class.
2) It allows you to treat a piece of functionality as a method argument or code as data.
3) A lambda expression can be passed around as if it were an object and executed on demand.
Modern Java - What’s new in Java 9 to Java 17
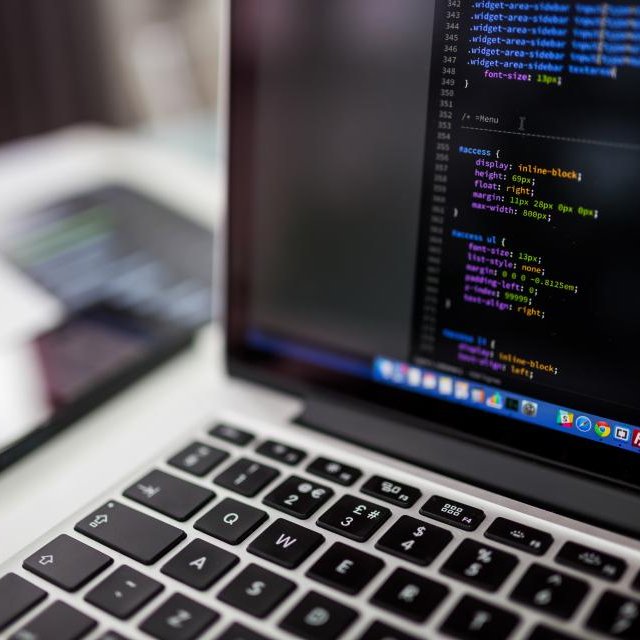
NK Chauhan
NK Chauhan is a Principal Software Engineer with one of the biggest E Commerce company in the World.
Chauhan has around 12 Yrs of experience with a focus on JVM based technologies and Big Data.
His hobbies include playing Cricket, Video Games and hanging with friends.